Table Of Content
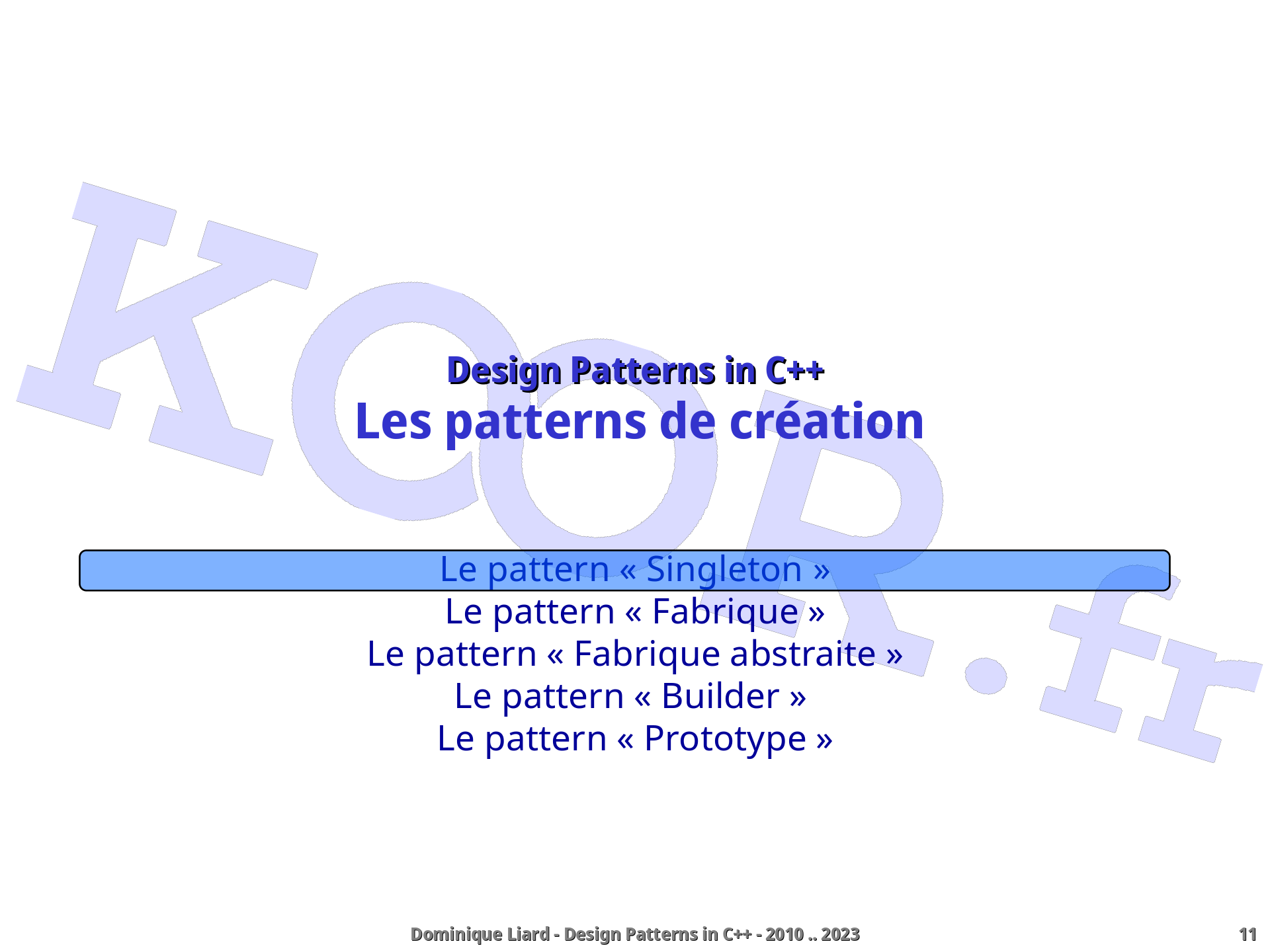
You can use this if you are sure you’ll use getInstance() at least one time in your application. In Factory Method,Pizza and Burger needed to be in type Meal,here it’s not compulsory. Factory Method helps you to select between pizza and burger by overriding a method but keeps non-varying parts in a common method. As your app grows larger, you may want to add new functionalities, such as weather information. Suppose you are building a social media app and implementing it for multiple platforms.
Extending The Factory
Creational design patterns solve the problems related to object creation. They help to abstract away object creation processes that spread across multiple classes. Design Patterns in Python introduces a powerful and essential concept in software development – design patterns. These patterns offer time-tested solutions to recurring design problems, providing a structured and efficient approach to building robust, scalable, and maintainable software systems in Python.
If it Looks Like a Duck, Quacks Like a Duck, But Needs Batteries - You Have the Wrong Abstraction - hackernoon.com
If it Looks Like a Duck, Quacks Like a Duck, But Needs Batteries - You Have the Wrong Abstraction.
Posted: Mon, 18 May 2020 07:00:00 GMT [source]
Factory Method Design Pattern
Unlike traditional inheritance, which can lead to a deep and inflexible class hierarchy, the decorator pattern uses composition. You can compose objects with different decorators to achieve the desired functionality, avoiding the drawbacks of inheritance, such as tight coupling and rigid hierarchies. The decorator pattern follows the open-closed principle, which states that classes should be open for extension but closed for modification. This means you can introduce new functionality to an existing class without changing its source code. The world of C programming is expansive, and design patterns act as guiding beacons for developers, aiding them in writing efficient and maintainable code. Let's delve into the benefits of incorporating these design patterns into your C projects.
Function Design Pattern
Intent of Memento Design pattern is without violating encapsulation, capture and externalize an object’s internal state so that the object can be restored to this state later. Be aware that you may violate the Open-Closed Principle.OCD basically says that classes should be open for extension, but closed for modification. Let’s say you have a code block like this where you let your client choose a frame for their photo.
Memento Method Design Patterns
To understand better, let's implement a factory for creating different types of products. By understanding and applying such patterns, you not only develop efficient code but also ensure your code stands the test of time. Always consider the context and the problem at hand before choosing a pattern to implement. The class is also sealed to prevent inheritance, which could lead to subclassing that breaks the singleton rules.

thoughts on “Design Patterns in C# With Real-Time Examples”
Assuming slices is a required parameter and the rest of them is not,it wouldn’t be wise to create a different constructor for each optional field to satisfy all object combinations. Singleton Pattern vs Dependency InjectionIf you are already using a framework that handles injections, it may be a better practice to just inject classes instead of applying Singleton Pattern. We can lock the getInstance() by using synchronized keyword and force other threads to wait current thread to exit before entering this code block. Remember to combine multiple patterns if you feel like it’ll be a good fit.
I prefer to first check if it’s a helper method, if yes, create a static class.If not, check our DI availabilities. Using this approach, we pass the responsibility to JVM and it initializes our Singleton instance when the class is loaded, so multithreading is not an issue. If one day you want to remove fur from the tabby for your game, you can just change how displayBehavior is set in the Tabby class and the client won’t need to be notified of this change. Design patterns differ by their complexity, level ofdetail and scale of applicability.
The classes, objects, and interfaces used in the above UML diagram are described below. Facade Method Design Pattern provides a unified interface to a set of interfaces in a subsystem. Facade defines a high-level interface that makes the subsystem easier to use. Abstract Factory pattern is almost similar to Factory Pattern and is considered as another layer of abstraction over factory pattern.
Simple Factory
If both Meals have a common method regardless of their type, you can turn MealFactory to abstract class instead of an interface. Loosely coupled designs allow us to build flexible object-oriented systems that can handle change. You can even write your own decorator to your program by simply extending the java.io package decorators’ superclass (FilterInputStream) and wrap it around the base class FileInputStream. Again, if one day you want to change the way how PostgreStrategy or CouchbaseStrategy works, you won’t need to touch the client code.
Creational design patterns are a subset of design patterns in software development. They deal with the process of object creation, trying to make it more flexible and efficient. It makes the system independent and how its objects are created, composed, and represented. The Factory Method pattern allows subclasses to choose the type of objects to create without revealing the creation process to the client. It provides an interface for creating objects in a way that is convenient and flexible for software development. The Singleton Pattern is one of the simplest design patterns in software development.
This is particularly useful when you need to adapt an object’s behavior based on changing requirements or user preferences. If you find your implementation too convoluted, revisit and refactor. Sometimes, it's imperative to have only one instance of a structure, especially when dealing with shared resources like configurations, logging, or connection pools. Singleton ensures that a system operates optimally without the overhead of instantiating a particular structure multiple times.
No comments:
Post a Comment